As already mentioned,
Jim's software is written such that it detects a change in pitch and roll (≥
2 degrees different from previous read cycle) and then switches a relay that
will drive the platform motor to get the platform moving in the direction of
the change. As soon as the pitch / roll change is less that 2 degrees, the
motor is switched off, and the platform will automatically return to center.
This method actually takes the derivative of the pitch / roll movement
(change per time unit), which is a measure for rotational acceleration.
I have done experiments based on the same principle,
sending the difference data to the
Parallel Port DAC interface.
When using the CHComm utility:
20 ON ERROR GOTO 140
30 CLOSE #1
40 OPEN "com1:9600,n,8" FOR RANDOM AS #1
50 A$=""
55 ON ERROR GOTO 50
60 LINE INPUT#1, A$
70 R=VAL(RIGHT$(A$,4))
80 P=VAL(LEFT$(A$,5))
81 PD=P-PL
82 RD=R-RL
90 DAC1=2*R+127
94 DAC2=6*RD+127
95 PRINT P;R;PL;RL;PD;RD;DAC1;DAC2
100 IF DAC1>255 or DAC1<0 then goto 115
110 OUT 888,DAC1
111 OUT 890,3
112 OUT 890,11
115 IF DAC2>255 or DAC2<0 then goto 120
116 OUT 888,DAC2
117 OUT 890,9
118 OUT 890,11
120 PL=P
121 RL=R
130 GOTO 50
140 RESUME 20
In the above program, RD and PD are the difference between current and
last reading
The RD and PD difference data are then send to the parallel port for
driving the DAC's. They
need to be scaled, and offset has to be applied to get the 0 degrees
in the center of the DAC range (128).
With regard to range, some
choices have to be made. You have to make sure that you don't exceed +127 or
– 127 from center value, or you will send an illegal value (< 0 or > 255) to
the parallel port. An error message will result.
If you plan to fly the Extra 300s, the pitch and roll rate can be pretty
fast. Large difference values like 30 or 40 degrees per read cycle will be
possible. If you fly the heavies, you will hardly reach 5 degrees difference
per read cycle. You may need to tweak your gain (DAC change per degree)
accordingly.
In the program, I have send actual roll data on one DAC
channel and the roll difference data on the 2nd DAC channel, just to make
the relation between the two visible.
The plot above shows the output of DAC1 (roll
data, upper waveform) and DAC2 (roll change, lower waveform). In flight,
this was the turn from base leg to final and touchdown, flying a Baron 58.
As you can see, the lower waveform very accurately shows the changes in roll
rate. Also the left-wheel first touchdown will result in a small bump.
(Horizontal scale 5sec / division)
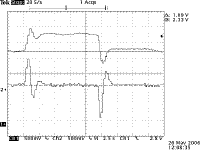
Another plot I made while doing a quick left
and a right turn flying the Extra. As you can see, the turn rate is much
faster, resulting in higher change spikes.
For fast aerobatics, the interface speed may be somewhat slow, as you can
see on the above plot with faster time scale. (2.5sec/division). The 0.2sec
update speed will result in staircasing in the response.
When using the Portdrv utility:
Pitch and Roll difference calculation can be done similar as with CHComm.
However, due to the faster update speed of Portdrv utility, this also
results in very small differences between new reading and last reading which
is needed for acceleration drive. Most "normal" flying would always result
in 0's as the result of New – Last reading. This makes sense as the shorter
time samples during the same roll change slope will result in smaller roll
change.
To solve this problem I have made the program look at more than one previous
reading. In this way you can compare the new reading with the last, one
before last, etc. In this way more gradual changes are also detected and can
be used set the output DAC accordingly:
88 P1=P2:P2=P3:P3=P4:P4=P5:P5=P
90 IF ABS(P5-P4)<1 THEN GOTO 91 ELSE PD=4*(P5-P4):GOTO
95 :REM biggest change
91 IF ABS(P5-P3)<1 THEN GOTO 92 ELSE PD=2*(P5-P3):GOTO 95 :REM smaller
change
92 IF ABS(P5-P2)<1 THEN GOTO 93 ELSE PD=INT(1.5*(P5-P2)):GOTO 95
93 IF ABS(P5-P1)<1 THEN PD=0 ELSE PD=P5-P1 : REM smallest change
The complete program is shown below:
20 ON ERROR GOTO 140
30 CLOSE #1
40 OPEN "com1:9600,n,8" FOR RANDOM AS #1
50 A$=""
55 ON ERROR GOTO 50
60 LINE INPUT#1, A$
70 P$=mid$(A$,3,2)
80 R$=mid$(A$,5,2)
85 P=INSTR("123456789ABCDEF",right$(P$,1))+16*INSTR("123456789ABCDEF",left$(P$,1))
86 R=INSTR("123456789ABCDEF",right$(R$,1))+16*INSTR("123456789ABCDEF",left$(R$,1))
88 P1=P2:P2=P3:P3=P4:P4=P5:P5=P
89 R1=R2:R2=R3:R3=R4:R4=R5:R5=R
90 IF ABS(P5-P4)<1 THEN GOTO 91 ELSE PD=4*(P5-P4):GOTO 95
91 IF ABS(P5-P3)<1 THEN GOTO 92 ELSE PD=2*(P5-P3):GOTO 95
92 IF ABS(P5-P2)<1 THEN GOTO 93 ELSE PD=INT(1.5*(P5-P2)):GOTO 95
93 IF ABS(P5-P1)<1 THEN PD=0 ELSE PD=P5-P1
95 IF ABS(R5-R4)<1 THEN GOTO 96 ELSE RD=4*(R5-R4):GOTO 100
96 IF ABS(R5-R3)<1 THEN GOTO 97 ELSE RD=2*(R5-R3):GOTO 100
97 IF ABS(R5-R2)<1 THEN GOTO 98 ELSE RD=INT(1.5*(R5-R2)):GOTO 100
98 IF ABS(R5-R1)<1 THEN RD=0 ELSE RD=R5-R1
100 DAC1=R
102 DAC2=3*RD+127
108 PRINT R1;" "R2;" "R3;" "R4;" "R5;" "RD;" "DAC1;" "DAC2
109 IF DAC1>255 or DAC1<0 then goto 117
110 OUT 888,DAC1
115 OUT 890,3
116 OUT 890,11
117 IF DAC2>255 or DAC2<0 then goto 130
118 OUT 888,DAC2
119 OUT 890,9
120 OUT 890,11
130 GOTO 50
140 RESUME 20
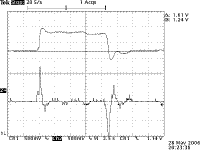
With this program I did the same maneuver in the Extra300s as previously
shown. The plot shows again roll data and the difference data. As can be
seen, the output is much faster, and differential signal is much sharper and
more dynamic.
The software based on above principle was finalized for driving both
pitch and roll:
20 ON ERROR GOTO 140
30 CLOSE #1
40 OPEN "com1:9600,n,8" FOR RANDOM AS #1
50 A$=""
55 ON ERROR GOTO 50
60 LINE INPUT#1, A$
70 P$=mid$(A$,3,2)
80 R$=mid$(A$,5,2)
85 P=INSTR("123456789ABCDEF",right$(P$,1))+16*INSTR("123456789ABCDEF",left$(P$,1))
86 R=INSTR("123456789ABCDEF",right$(R$,1))+16*INSTR("123456789ABCDEF",left$(R$,1))
88 P1=P2:P2=P3:P3=P4:P4=P5:P5=P
89 R1=R2:R2=R3:R3=R4:R4=R5:R5=R
90 IF ABS(P5-P4)<1 THEN GOTO 91 ELSE PD=4*(P5-P4):GOTO 95
91 IF ABS(P5-P3)<1 THEN GOTO 92 ELSE PD=2*(P5-P3):GOTO 95
92 IF ABS(P5-P2)<1 THEN GOTO 93 ELSE PD=INT(1.5*(P5-P2)):GOTO 95
93 IF ABS(P5-P1)<1 THEN PD=0 ELSE PD=P5-P1
95 IF ABS(R5-R4)<1 THEN GOTO 96 ELSE RD=4*(R5-R4):GOTO 100
96 IF ABS(R5-R3)<1 THEN GOTO 97 ELSE RD=2*(R5-R3):GOTO 100
97 IF ABS(R5-R2)<1 THEN GOTO 98 ELSE RD=INT(1.5*(R5-R2)):GOTO 100
98 IF ABS(R5-R1)<1 THEN RD=0 ELSE RD=R5-R1
100 DAC1=4*PD+2*P-127
102 DAC2=4*RD+127
108 PRINT R;" "P;" "RD;" "PD;" "DAC2;" "DAC1
109 IF DAC1>255 or DAC1<0 then goto 117
110 OUT 888,DAC1
115 OUT 890,3
116 OUT 890,11
117 IF DAC2>255 or DAC2<0 then goto 130
118 OUT 888,DAC2
119 OUT 890,9
120 OUT 890,11
130 GOTO 50
140 RESUME 20
First trials with this software driving the platform: See Test Flight video.
There are some drawbacks: